View the ADU series of USB based Data Acquisition Products
Introduction
Communicating with USB devices in Visual Studio, or
virtually any application software, involves a few
simple steps. Unlike RS232 based devices which are
connected to physical COM ports, USB devices are
assigned a logical handle
by operating systems when they are first plugged in.
This process is known as enumeration.
Once a USB device has been
enumerated, it is ready for use by the host computer
software. For the host application software to
communicate with the USB device, it must first obtain
the handle assigned to the USB device during the
enumeration process. The handle can be obtained using an
open function along with
some specific information about the USB device.
Information that can be used to obtain a handle to a USB
device include, serial number,
product ID, or vendor
ID. Once the handle is obtained, it is used to allow the
application to read and write information, to and from,
the USB device. Once the application has finished
with all communication with the USB device, the handle
is closed. The handle is generally closed when the
application terminates.
The AduHid DLL provides all the
functions to open a handle, read and write data, and
close the handle of ADU USB devices. The ADUHID dll can
be used directly from a Visual Basic .NET application
including Visual Studio 2015.
The sample program below is a
rendition of our AduHidTest program demonstrating the proper method to
utilize the functions within the ADUHID dll to
communicate with ADU, USB based data acquisition
products. The
example also includes use of the functions ADUCount,
GetADU and GetAduDeviceList which are new to the ADUHID
and ADUHID64 dll's Ver 2.1.
The resulting executable was tested under Windows 7 and 10.
All source code is provided so that you may review details that
are not highlighted here.
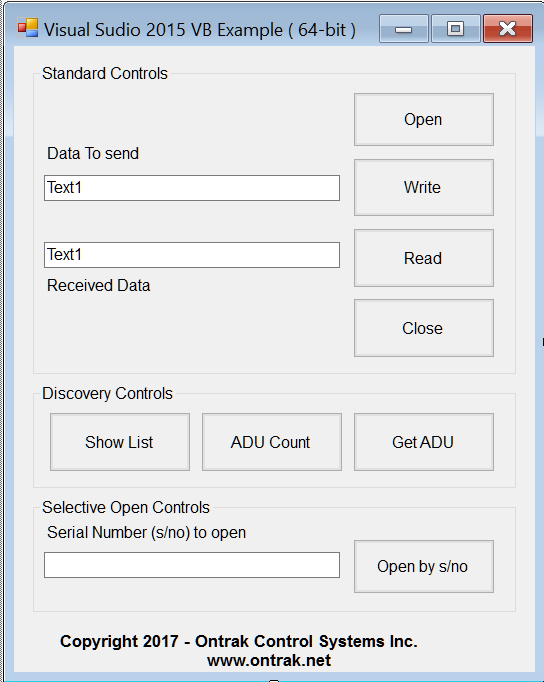
Figure
1. AduHidTest Visual Studio 2015 VB version
IMPORTANT:
The sample program provided
requires access to the ADUHID DLL. To enable this
you should run the Visual Studio environment with Administrator
privileges. To open Visual Studio 2015 with
Administrator privileges, right click the program icon and select :Run As Administrator.
See Figure 2.
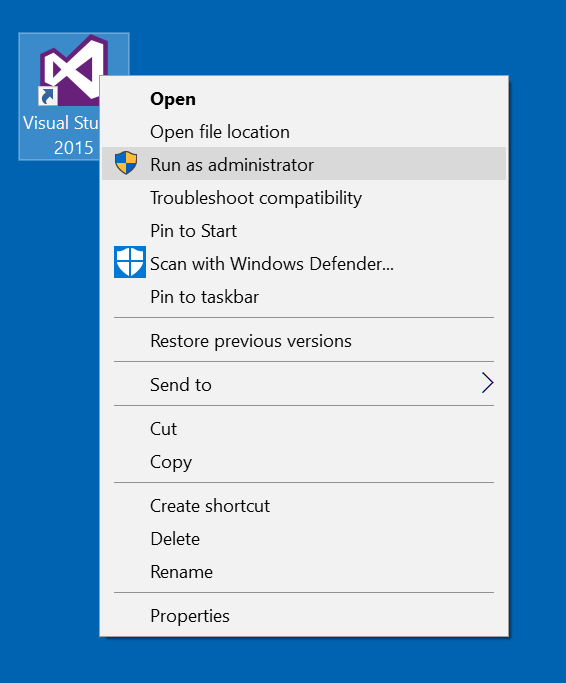
Figure
2: Starting Visual Studio 2015 using Right Click to Run as
administrator
Lets have a look at the code......
For Visual Studio to have access to the functions inside
the AduHid dll the individual functions must be declared
outlining the syntax and data formats used by each
function.
Module1 in our program declares all the functions to be
called by the application. In the example shown, the
AduHid64.dll is referenced. Note that in the 32 bit
version, the AduHid.dll ( 32 bit version) is referenced.
Note that specific details of the individual
functions are available in the
ADU On-line SDK.
(Small text used to make it fit the web page....full
size text in the sample code download below !)
Option Strict Off
Option Explicit On
Module Module1
Structure ADU_DEVICE_ID
Dim iVendorId As Short
Dim iProductId As Short
<VBFixedString(7),
System.Runtime.InteropServices.MarshalAs(System.Runtime.InteropServices.UnmanagedType.ByValTStr,
SizeConst:=7)> Public sSerialNumber As String
End Structure
Declare Function OpenAduDevice Lib "AduHid64.DLL" (ByVal
iTimeout As Integer) As Integer
Declare Function WriteAduDevice Lib "AduHid64.DLL"
(ByVal aduHandle As Integer, ByVal lpBuffer As String,
ByVal lNumberOfBytesToWrite As Integer, ByRef
lBytesWritten As Integer, ByVal iTimeout As Integer) As
Integer
Declare Function ReadAduDevice Lib "AduHid64.DLL" (ByVal
aduHandle As Integer, ByVal lpBuffer As String, ByVal
lNumberOfBytesToRead As Integer, ByRef lBytesRead As
Integer, ByVal iTimeout As Integer) As Integer
Declare Function CloseAduDevice Lib "AduHid64.DLL"
(ByVal iOverlapped As Integer) As Integer
Declare Function ShowAduDeviceList Lib "AduHid64.DLL"
(ByRef pAduDeviceId As ADU_DEVICE_ID, ByVal sPrompt As
String) As Integer
Declare Function OpenAduDeviceBySerialNumber Lib
"AduHid64.DLL" (ByVal pSerialNumber As String, ByVal
iTimeout As Integer) As Integer
Declare Function ADUCount Lib "AduHid64.DLL" (ByVal
iTimeout As Integer) As Integer
Declare Function GetADU Lib "AduHid64.DLL" (ByRef
pAduDeviceId As ADU_DEVICE_ID, ByVal iIndex As Integer,
ByVal iTimeout As Integer) As Integer
End Module
The Standard Controls
The Standard Controls, function identical to the
AduHidTest software. There are four buttons available to
Open, Write,
Read, and Close,
and, two text boxes for Data To Send
and Received Data. The OPEN
button retrieves the handle for the first ADU device it
sees using the Ontrak Vendor ID. If you have more
than one ADU connected, see below section on Selective
Open Controls.
Once the handle is obtained, the command to send to
the ADU is typed into the Data To Send
text box, and the Write button
is pressed to send the command to the ADU. If the
command is sending a response, the Read
button can now be activated and the data read back will
be displayed in the Received Data
text box. The Close button can
then be pressed to close the handle to the ADU.
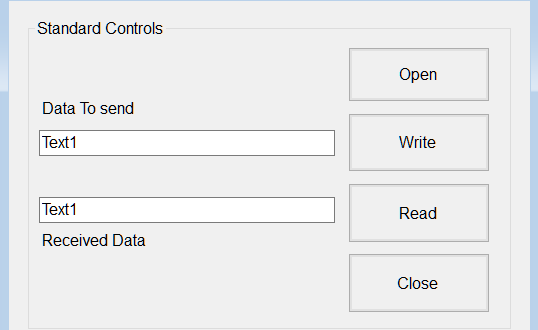
Figure 3: The Standard Controls
Here are the subroutines
for each element and a few
comments.
The Open button
Dim aduHandle As Integer
Private Sub cmdOpen_Click(ByVal eventSender As
System.Object, ByVal eventArgs As System.EventArgs)
Handles cmdOpen.Click
aduHandle = OpenAduDevice(1)
'Here we get a handle to
the ADU device connected via the USB port
End Sub
The Write button
Private Sub cmdWrite_Click(ByVal eventSender As
System.Object, ByVal eventArgs As System.EventArgs)
Handles cmdWrite.Click
Dim iRC As Integer
Dim iBytesWritten As Integer
iRC = WriteAduDevice(aduHandle, txtCommand.Text,
Len(txtCommand.Text), iBytesWritten, 500)
'txtCommand.Text is whatever data has been typed into
the Data To Send text box.
End Sub 'timout
is set to 500ms
The Read button
Private Sub cmdRead_Click(ByVal eventSender As
System.Object, ByVal eventArgs As System.EventArgs)
Handles cmdRead.Click
Dim iRC As Integer
Dim iBytesRead As Integer
Dim sResponse As String
sResponse = "+++No Data+++"
' sresponse is preloaded to set its size and show +++No
Data+++ if there is no data!
iRC = ReadAduDevice(aduHandle, sResponse, 7, iBytesRead,
500)
txtResponse.Text = sResponse
'Data is displayed in Received Data textbox.
End Sub
The Close button
Private Sub cmdClose_Click(ByVal eventSender As
System.Object, ByVal eventArgs As System.EventArgs)
Handles cmdClose.Click
CloseAduDevice(aduHandle) ' Here we close the handle to
the ADU device connected to the USB port
aduHandle = 0
End Sub
The Discovery Controls
The Discovery Controls are advanced functions built
into the AduHid dll to obtain information about ADU
devices connected to the host computer. These
functions can be used to automate the selection of
connected ADU devices. The Show List
button causes a pop-up window to display a list of
connected devices and some relevant information. The
ADU Count button causes a
message box to appear showing how many ADU devices are
connected. The Get ADU button
causes a message box to display the connected devices
one at a time including their index number.
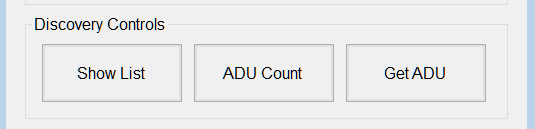
Figure 4: The Discovery Controls
Here are the subroutines
for each element and a few
comments.
The Show List button
Private Sub cmdShowList_Click(ByVal eventSender As
System.Object, ByVal eventArgs As System.EventArgs)
Handles cmdShowList.Click
Dim myAduDeviceId As ADU_DEVICE_ID
Dim iRC As Integer
iRC = ShowAduDeviceList(myAduDeviceId, "Connected ADU
Devices")
'triggers the pop-up window
End Sub
The ADU Count button
Private Sub cmdADUCount_Click(ByVal sender As
System.Object, ByVal e As System.EventArgs) Handles
cmdADUCount.Click
MsgBox("ADU Count: " & ADUCount(100))
' count is limited to
100 devices
End Sub
The GetADU button
Private Sub cmdGetADU_Click(ByVal sender As
System.Object, ByVal e As System.EventArgs) Handles
cmdGetADU.Click
Dim pADU As ADU_DEVICE_ID
For iAduIndex As Integer = 0 To ADUCount(100) - 1
GetADU(pADU, iAduIndex, 100)
MsgBox("Index: " & iAduIndex & " Model: " &
pADU.iProductId & " Serial Number: " &
pADU.sSerialNumber)
Next 'if multiple
devices present, next window pops up after each is
closed
End Sub
Selective Open Control
The Selective Open Control allows the handle to a
specific connected ADU device to be obtained by typing
the serial number into the Serial Number
(s/no) to open text box and clicking the
Open by s/no button. The
Standard Controls can then be used to communicate with
the selected ADU device.
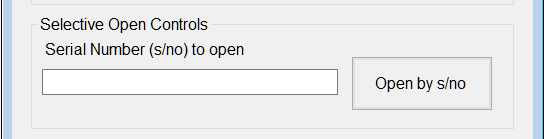
Figure 5: Selective
Open Control
Here is the
subroutine.
The Open by s/no button
Private Sub
cmdOpenBySNo_Click(ByVal sender As System.Object, ByVal
e As System.EventArgs) Handles cmdOpenBySNo.Click
aduHandle = OpenAduDeviceBySerialNumber(txtSNo.Text, 1)
End Sub
DOWNLOAD Visual Studio 2015 VB Example Files in
ZIP format for 32-Bit Development.
DOWNLOAD Visual Studio 2015 VB Example Files in
ZIP format for 64-Bit Development.
|